[affi id=2]
Laravel開発の自動化をしてみたいのでカスタムコマンドを作成してみたいと思います。
早速やっていきましょう。
カスタムコマンドを作成する
新しいコマンドを作成するには、
https://readouble.com/laravel/8.x/ja/artisan.htmlmake:command
Artisanコマンドを使用します。このコマンドは、app/Console/Commands
ディレクトリに新しいコマンドクラスを作成します。このディレクトリがアプリケーションに存在しなくても心配しないでください。make:command
Artisanコマンドを初めて実行したとき、このディレクトリを作成します。
ドキュメントに書いてある通り、make:command を使用して独自コマンドを作ります。
今回はテストのためのコマンドを作成したいので以下のコマンドを打ち込みましょう。
※TestCommandの部分は好きな名前にしてください。
php artisan make:command TestCommand
[Console command created successfully.]と表示されたら成功です。
[プロジェクトフォルダ]\app\Console\Commands 配下にTestCohmmand.phpが作成されているはずです。
作成されたファイルの内容
<?php
namespace App\Console\Commands;
use Illuminate\Console\Command;
class TestCommand extends Command
{
/**
* The name and signature of the console command.
*
* @var string
*/
protected $signature = 'command:name';
/**
* The console command description.
*
* @var string
*/
protected $description = 'Command description';
/**
* Create a new command instance.
*
* @return void
*/
public function __construct()
{
parent::__construct();
}
/**
* Execute the console command.
*
* @return int
*/
public function handle()
{
return 0;
}
}
[ad]
Hello Worldしてみよう
コマンドファイルができたら実行して「Hello World!」と出力させてみましょう。
コマンドを実行するための設定
コマンドを生成した後に、クラスの
https://readouble.com/laravel/8.x/ja/artisan.htmlsignature
プロパティとdescription
プロパティに適切な値を定義する必要があります。
ドキュメントにあるようにいくつかの設定をします。
① php artisan signatureプロパティ のように実行することになるので今回はここだけ修正します。
※descriptionプロパティはlistコマンドで一覧表示された時の説明文になるので今回は設定せずそのままの値にします。
signatureプロパティの設定
<?php
namespace App\Console\Commands;
use Illuminate\Console\Command;
class TestCommand extends Command
{
/**
* The name and signature of the console command.
*
* @var string
*/
//protected $signature = 'command:name'; ここを変更する。
protected $signature = 'test:command';
/**
* The console command description.
*
* @var string
*/
protected $description = 'Command description';
/**
* Create a new command instance.
*
* @return void
*/
public function __construct()
{
parent::__construct();
}
/**
* Execute the console command.
*
* @return int
*/
public function handle()
{
return 0;
}
}
今回はsignatureプロパティを"test:command"としました。こうすることにより以下のコマンドで実行することができます。
php artisan test:command
Hello Worldを表示させる
コマンドが実行できるようになったので実際に「Hello World!」を表示しましょう。
laravelのコマンドでは以下のようなメソッドでコンソールに文字を出力することができます。
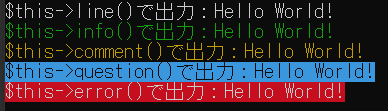
出力タイプ | 説明 |
---|---|
line | コンソールに通常のテキストを表示 |
info | コンソールに緑色のテキストを表示 |
comment | コンソールに黄色のテキストを表示 |
question | コンソールに青背景のテキストを表示 |
error | コンソールに赤背景のテキストを表示 |
Hello Worldを出力するソース
<?php
namespace App\Console\Commands;
use Illuminate\Console\Command;
class TestCommand extends Command
{
/**
* The name and signature of the console command.
*
* @var string
*/
//protected $signature = 'command:name'; ここを変更する。
protected $signature = 'test:command';
/**
* The console command description.
*
* @var string
*/
protected $description = 'Command description';
/**
* Create a new command instance.
*
* @return void
*/
public function __construct()
{
parent::__construct();
}
/**
* Execute the console command.
*
* @return int
*/
public function handle()
{
$this->line('$this->line()で出力:' . "Hello World!");
$this->info('$this->info()で出力:' . "Hello World!");
$this->comment('$this->comment()で出力:' . "Hello World!");
$this->question('$this->question()で出力:' . "Hello World!");
$this->error('$this->error()で出力:' . "Hello World!");
return 0;
}
}
それではコマンドを実行してみましょう!
php artisan test:command
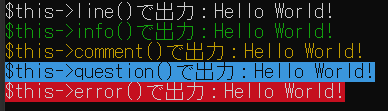
こんな感じで出力されたら成功です!お疲れさまでした。